Creating your own Dart package is a rewarding journey that allows you to solve problems, share solutions, and contribute to the growing Dart ecosystem. Whether you're looking to modularize your own code, open-source a useful utility, or build your reputation in the developer community, publishing a package on pub.dev gives your code a home where others can discover and use it.
In this tutorial, you'll learn the complete process of creating, testing, and publishing a Dart package. You'll discover how to structure your code properly, write effective documentation, implement thorough testing, and navigate the publishing process.
By the end of this blog, you'll have all the knowledge needed to add your own creation to the thousands of packages that make Dart and Flutter development so productive. Let's get started on transforming your code into a package that developers around the world can use in their projects.
Understanding Dart Packages
Dart packages are the fundamental building blocks of code reuse in the Dart ecosystem. They allow developers to share code, extend functionality, and build upon each other's work—creating a vibrant community of tools and libraries.
What is a Dart Package
At its core, a Dart package is a directory containing Dart code organized in a specific way, along with a pubspec.yaml
file that describes the package, its dependencies, and other metadata. This structured approach makes it easy for the Dart package manager (pub
) to manage dependencies, ensuring that the right versions of the right packages are used together.
Packages can contain libraries, executable programs, resources, tests, examples, and documentation. When you work with Dart or Flutter, you're constantly using packages—from the core libraries provided by the Dart SDK to third-party packages published on pub.dev.
Types of Packages: Application vs Library
Dart recognizes two primary types of packages:
Application Packages: These are meant to be directly executed and not imported by other packages. They typically include a bin
directory with executable Dart programs. Examples include command-line tools, servers, and entire Flutter applications.
Library Packages: These are designed to be imported and used by other Dart code. They provide reusable functionality through a well-defined API. When developers talk about "creating a Dart package," they're most often referring to library packages. The code in these packages lives primarily in the lib
directory.
Creating a Dart Package
Before you can publish your Dart package, you first need to create it. Dart provides built-in tools to generate a structured package quickly. In this section, we'll cover how to create a package using the Dart command-line tools and explore the basic structure of a Dart package.
Prerequisites
Before diving into creating your Dart package, you need to set up your development environment with the right tools.
These are a few things you must have in place to successfully complete the tutorial:
- You have installed the Dart SDK on your local machine.
- You have a code editor or IDE (e.g. VS Code, IntelliJ or Android Studio) that supports Dart programming language.
Creating your package directory structure
Once you have the required tools and setup done, you can create the structure for your new package:
- Open your terminal and navigate to your desired parent directory
- Create a new package with the Dart CLI:
dart create -t package <PACKAGE_NAME>
Remember to replace my_dart_package
with your desired package name. The -t package
flag ensures that Dart generates the correct structure for a reusable package rather than an application.
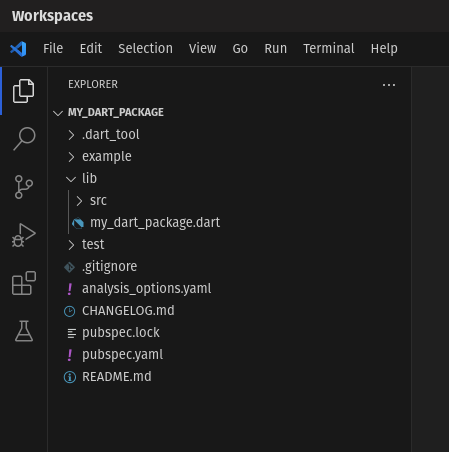
Structure of a Dart Package
After running this command, you’ll see a new directory created with the following structure:
my_dart_package/
├── .dart_tool/
├── .gitignore
├── lib/
│ ├── my_dart_package.dart
│ └── src/
├── test/
├── example/
├── pubspec.yaml
├── pubspec.lock
├── CHANGELOG.md
├── README.md
└── LICENSE
The most critical parts of this structure are:
pubspec.yaml
: Defines your package name, version, description, dependencies, and other metadata.lib/
: Contains the public API of your package, with implementation details often hidden in asrc
subdirectory.lib/my_dart_package.dart
: The main entry point that exports the public API of your package.test/
: This directory contains test files to validate the functionality of your package. Writing tests is essential to ensure reliability.README.md
: A markdown file where you provide an overview of your package, usage instructions, and examples.CHANGELOG.md
: This file tracks changes, updates, and improvements across different package versions.analysis_options.yaml
: This optional file allows you to enforce linting rules and best practices in your package.
Once your directory structure is in place, open the project in your preferred IDE and begin configuring your package details in the pubspec.yaml
file:
name: my_dart_package
description: A brief description of what your package does
version: 0.1.0
homepage: https://github.com/yourusername/my_dart_package
environment:
sdk: ">=3.0.0 <4.0.0"
dependencies:
# List external packages your package depends on
dev_dependencies:
lints: ^2.0.0
test: ^1.21.0
Understanding this structure is essential because it impacts how others will use your package and how effectively you can maintain it over time. A well-organized package makes it easier for users to understand your code and for you to add features or fix bugs without breaking existing functionality.
Implementing Your Package
Now that your package is set up, it's time to bring your package to life through actual code. This phase is where you can start writing code to give a working solution that delivers real value to users.
Writing the core functionality
Start by implementing the primary features of your package, focusing on the core functionality that solves the main problem you identified. Follow these principles for quality implementation:
- Start with interfaces: Define your class interfaces and method signatures before diving into implementations.
- Separate concerns: Keep different responsibilities in different classes or libraries.
- Hide implementation details: Place internal code in the
lib/src
directory and only expose what's needed through your main library file. - Error handling: Provide clear error messages and appropriate exception types.
- Performance considerations: Be mindful of memory usage and computational efficiency, especially for operations that might be called frequently.
Here's a simple example of how to structure your implementation:
Filename - lib/src/calculator.dart
// lib/src/calculator.dart - Implementation
import 'models/calculation_result.dart';
class Calculator {
/// A simple utility method that adds two numbers.
CalculationResult add(double a, double b) {
return CalculationResult(a + b);
}
// Additional methods...
}
Exporting Additional Files
If you plan to add more functionality and organize your package into multiple files, you should create separate Dart files inside the lib/
directory and export them in your main lib/my_dart_package.dart
file.
Filename - lib/utils.dart
/// A simple utility function that multiplies two numbers.
int multiply(int a, int b) {
return a * b;
}
Then, update lib/my_dart_package.dart
to export the lib/src/calculator.dart
and lib/utils.dart
and other files:
Filename - lib/my_dart_package.dart
// lib/my_dart_package.dart - Public API
library my_dart_package;
export 'src/calculator.dart';
export 'src/models/calculation_result.dart';
export 'utils.dart'
Testing Your Package
Testing is a crucial part of package development to ensure reliability and correctness. Writing tests helps catch bugs early, improves code quality, and makes it easier to maintain your package over time.
Unit Testing with test Package
Unit tests verify that individual components of your package work as expected in isolation. The Dart test
package provides a robust framework for writing and running these tests.
Start by adding the test package to your dev dependencies in pubspec.yaml
:
dev_dependencies:
test: ^1.21.0
Create test files in the test/
directory, following these best practices:
- Name test files with a
_test.dart
suffix - Group related tests using the
group()
function - Write descriptive test names that explain the expected behavior
- Use
setUp()
andtearDown()
for common initialization and cleanup
More notes on unit testing with the test
package is covered in this tutorial.
Running Tests
To execute all tests, run the following command in the terminal:
dart test
Code Coverage
Code coverage measures how much of your code is exercised by your tests. High coverage helps ensure comprehensive testing and reduces the risk of undiscovered bugs.
To analyze code coverage in your Dart package:
- Run your tests with coverage enabled:
dart run test --coverage=coverage
- Generate a coverage report:
dart run coverage:format_coverage --lcov --in=coverage --out=coverage/lcov.info --packages=.packages --report-on=lib
- For a visual representation, convert the report to HTML (requires lcov tool):
genhtml coverage/lcov.info -o coverage/html
Aim for high coverage (80%+) but remember that coverage alone doesn't guarantee quality tests. Focus on meaningful assertions that verify correct behavior rather than simply executing code.
Documenting Your Package
Creating and maintaining good documentation is essential for making your Dart package maintainable and easy to use. A well-documented package helps other developers understand its functionality, reduces confusion, and increases adoption.
README.md files
The README.md
file is the first thing users see when discovering your package. It serves as both an introduction and a quick reference guide. A good package README.md
file should include:
- Package Name and Description: This is a concise overview of your package and what it does.
- Installation Instructions: This explains how to add your package to a Dart or Flutter project.
- Basic Usage and Guide: Simple examples showing basic usage.
- Features List: Highlight key functionalities.
- Contributing Guidelines – Instructions for contributing (optional but recommended).
- License Information: How others can use and distribute your code.
Creating a CHANGELOG
A CHANGELOG.md file tracks the evolution of your package and helps users understand what changes to expect when updating.
For each version, document:
- New features
- Bug fixes
- Breaking changes
- Deprecations
- Performance improvements
Structure your changelog with the most recent version at the top and follow a consistent format:
# Changelog
## 1.1.0
* Added: New `formatDate()` method for timestamp formatting
* Fixed: Bug in `capitalize()` that caused errors with empty strings
* Improved: Performance of string processing by 30%
## 1.0.1
* Fixed: Package description and metadata
## 1.0.0
* Initial stable release
* Added core formatting functionality
Follow semantic versioning principles when updating your CHANGELOG, making it clear which changes are breaking vs. compatible.
Well-crafted documentation is an investment that pays off in user adoption, reduced support requests, and smoother contribution experiences. Take the time to document thoroughly as you develop, rather than treating it as an afterthought.
Publishing Your Package
After you have finished developing, testing, and documenting your Dart package, the final step is to publish it to pub.dev, Dart's official package repository. This allows other developers to easily discover and use your package.
Follow the listed steps to complete the publication of your Dart package:
1. Create a pub.dev Account
Before you can publish a package, you need an account on pub.dev:
- Visit pub.dev and click on "Sign in" in the top-right corner.
- Sign in with a Google account.
- Review and accept the terms of service.
For packages published under an organization name, you'll want to create or join a publisher:
- Go to your profile page after signing in
- Navigate to the "Publishers" tab
- Create a new publisher or request to join an existing one
- Verify ownership of the domain associated with your publisher
Using a verified publisher adds credibility to your packages and groups them together on pub.dev.
2. Preparing Your Package for Publication
Before publishing, make sure your package meets these requirements:
- Correct
pubspec.yaml
setup: Ensure your package has a name, version, description, and dependencies listed properly. - Well-documented code: Use DartDoc comments and update
README.md
,CHANGELOG.md
, andLICENSE
. - Meaningful versioning: Follow Semantic Versioning (semver).
- No analysis issues: Run
dart analyze
to ensure there are no errors or warnings. - Comprehensive tests: Ensure your package has sufficient test coverage with
dart test
.
3. Run Pre-Publish Checks
Dart provides a command to verify that your package is ready for publication:
dart pub publish --dry-run
This performs checks such as:
- Ensuring the package name is valid
- Checking for missing or invalid metadata
- Verifying that dependencies are correctly defined
If any issues are found, fix them before proceeding.
4. Publishing to pub.dev
Once everything is ready, publish your package using:
dart pub publish
You’ll be prompted to confirm the publication. Type y
and press Enter to proceed.
If this is your first time publishing, you'll need to authenticate with your Google account. Follow the instructions in the terminal.
After successful publication, your package will be available at:
https://pub.dev/packages/YOUR_PACKAGE_NAME
Conclusion
Creating and publishing your own Dart package is both satisfying and valuable that allows you to contribute to the developer community while improving your own coding skills. By following a structured approach—setting up your package, writing clean and modular code, adding dependencies, testing thoroughly, documenting properly, and finally publishing to pub.dev
—you ensure that your package is useful, maintainable, and easy to adopt.
With your package live, continue refining it by addressing user feedback, adding new features, and keeping it up to date.